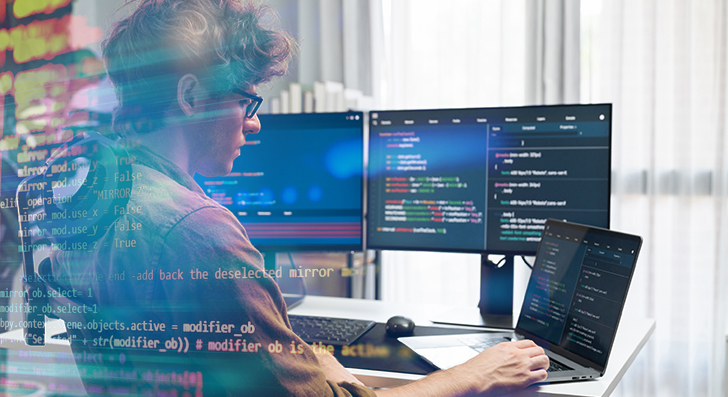
Scalability implies your software can cope with progress—much more buyers, additional knowledge, and even more visitors—without breaking. To be a developer, constructing with scalability in mind will save time and strain later on. Here’s a transparent and useful manual to help you start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not some thing you bolt on afterwards—it should be aspect of one's approach from the beginning. Lots of programs are unsuccessful if they expand speedy due to the fact the first design and style can’t tackle the extra load. For a developer, you have to Assume early about how your procedure will behave under pressure.
Start off by creating your architecture to get adaptable. Steer clear of monolithic codebases wherever every thing is tightly related. Rather, use modular layout or microservices. These patterns break your application into lesser, independent elements. Just about every module or service can scale By itself without the need of affecting The entire technique.
Also, give thought to your database from day a single. Will it will need to take care of 1,000,000 people or simply just a hundred? Choose the proper variety—relational or NoSQL—based upon how your details will grow. Strategy for sharding, indexing, and backups early, Even when you don’t have to have them yet.
An additional crucial position is to stop hardcoding assumptions. Don’t produce code that only is effective under current problems. Contemplate what would materialize When your consumer base doubled tomorrow. Would your app crash? Would the database slow down?
Use style styles that guidance scaling, like information queues or celebration-pushed programs. These support your app manage a lot more requests without having acquiring overloaded.
Any time you Establish with scalability in your mind, you're not just getting ready for success—you're reducing future problems. A perfectly-planned system is less complicated to take care of, adapt, and improve. It’s superior to arrange early than to rebuild later on.
Use the correct Database
Deciding on the correct databases is usually a critical Component of making scalable purposes. Not all databases are crafted the same, and utilizing the Erroneous one can sluggish you down and even trigger failures as your app grows.
Get started by comprehension your info. Is it highly structured, like rows inside of a desk? If yes, a relational database like PostgreSQL or MySQL is a great suit. They're powerful with interactions, transactions, and consistency. They also guidance scaling approaches like study replicas, indexing, and partitioning to take care of extra traffic and data.
In the event your information is a lot more adaptable—like consumer activity logs, merchandise catalogs, or paperwork—think about a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing huge volumes of unstructured or semi-structured details and may scale horizontally extra very easily.
Also, take into consideration your go through and create designs. Are you currently executing plenty of reads with less writes? Use caching and skim replicas. Are you currently dealing with a major create load? Investigate databases that will cope with high compose throughput, or maybe party-based info storage devices like Apache Kafka (for non permanent data streams).
It’s also intelligent to Consider ahead. You may not want State-of-the-art scaling options now, but deciding on a database that supports them indicates you won’t want to change later on.
Use indexing to hurry up queries. Avoid needless joins. Normalize or denormalize your knowledge determined by your entry styles. And generally observe databases general performance when you mature.
In brief, the correct database is determined by your app’s structure, speed needs, And exactly how you hope it to mature. Choose time to select correctly—it’ll help you save loads of issues later on.
Enhance Code and Queries
Quickly code is key to scalability. As your application grows, each individual smaller hold off adds up. Poorly composed code or unoptimized queries can slow down performance and overload your system. That’s why it’s imperative that you Make productive logic from the start.
Start by crafting cleanse, basic code. Stay away from repeating logic and remove anything at all unnecessary. Don’t pick the most intricate Remedy if a simple just one performs. Keep your capabilities quick, focused, and simple to check. Use profiling resources to find bottlenecks—destinations in which your code takes far too extended to operate or makes use of too much memory.
Upcoming, examine your databases queries. These generally slow matters down over the code alone. Be sure each query only asks for the info you actually have to have. Stay away from Find *, which fetches every little thing, and rather pick out specific fields. Use indexes to speed up lookups. And stay clear of carrying out a lot of joins, Particularly throughout large tables.
Should you see exactly the same facts being asked for many times, use caching. Shop the final results quickly utilizing equipment like Redis or Memcached this means you don’t need to repeat pricey functions.
Also, batch your databases functions when you can. As opposed to updating a row one by one, update them in groups. This cuts down on overhead and helps make your application additional efficient.
Remember to check with massive datasets. Code and queries that get the job done great with 100 records may well crash whenever they have to handle 1 million.
In brief, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when essential. These techniques assistance your software continue to be sleek and responsive, at the same time as the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to deal with a lot more consumers and a lot more website traffic. If anything goes by a person server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. These two tools help keep the application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Rather than one server doing many of the do the job, the load balancer routes people to distinctive servers dependant on availability. What this means is no solitary server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other individuals. Resources like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to create.
Caching is about storing information quickly so it might be more info reused promptly. When consumers ask for the exact same data again—like an item webpage or a profile—you don’t should fetch it from your databases whenever. You are able to provide it from your cache.
There's two prevalent varieties of caching:
one. Server-aspect caching (like Redis or Memcached) suppliers knowledge in memory for fast entry.
two. Consumer-facet caching (like browser caching or CDN caching) merchants static files near to the person.
Caching decreases database load, increases speed, and would make your app far more efficient.
Use caching for things that don’t improve usually. And normally be sure your cache is updated when knowledge does change.
Briefly, load balancing and caching are simple but highly effective instruments. With each other, they assist your application cope with a lot more users, remain speedy, and Recuperate from challenges. If you plan to expand, you would like each.
Use Cloud and Container Instruments
To make scalable programs, you will need instruments that let your app expand quickly. That’s where cloud platforms and containers are available in. They provide you overall flexibility, cut down set up time, and make scaling much smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and solutions as you will need them. You don’t really need to obtain components or guess upcoming potential. When traffic increases, you are able to include a lot more sources with only a few clicks or instantly employing automobile-scaling. When targeted traffic drops, you may scale down to save money.
These platforms also offer services like managed databases, storage, load balancing, and security tools. You can deal with setting up your application as an alternative to taking care of infrastructure.
Containers are One more crucial Instrument. A container packages your application and anything it should run—code, libraries, settings—into a person unit. This can make it uncomplicated to move your app concerning environments, from a laptop computer for the cloud, with out surprises. Docker is the preferred Resource for this.
Whenever your app works by using a number of containers, resources like Kubernetes help you regulate them. Kubernetes handles deployment, scaling, and recovery. If 1 element of your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate aspects of your app into services. You may update or scale elements independently, which happens to be perfect for overall performance and trustworthiness.
In a nutshell, utilizing cloud and container instruments implies you could scale speedy, deploy simply, and Get better speedily when issues transpire. If you'd like your application to develop devoid of limitations, start out utilizing these equipment early. They save time, minimize hazard, and enable you to keep focused on setting up, not repairing.
Watch Anything
Should you don’t observe your application, you received’t know when issues go Completely wrong. Monitoring will help the thing is how your app is doing, location problems early, and make greater conclusions as your app grows. It’s a important Section of setting up scalable methods.
Commence by monitoring fundamental metrics like CPU utilization, memory, disk Place, and reaction time. These tell you how your servers and companies are executing. Tools like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this data.
Don’t just check your servers—keep an eye on your application much too. Keep an eye on how long it will take for consumers to load webpages, how often problems take place, and the place they arise. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for vital complications. Such as, In the event your reaction time goes higher than a Restrict or possibly a provider goes down, you must get notified right away. This aids you repair problems fast, often right before people even detect.
Monitoring can also be valuable once you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you could roll it back again prior to it results in authentic injury.
As your app grows, website traffic and knowledge improve. Without the need of monitoring, you’ll miss indications of problems until it’s far too late. But with the correct tools set up, you remain in control.
Briefly, monitoring can help you keep your application dependable and scalable. It’s not pretty much spotting failures—it’s about being familiar with your technique and making sure it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for large corporations. Even little applications need a robust Basis. By developing diligently, optimizing properly, and utilizing the right equipment, you could Make apps that expand effortlessly with out breaking stressed. Get started tiny, Assume big, and Construct clever.